How to create Scalable Applications for a Developer By Gustavo Woltmann
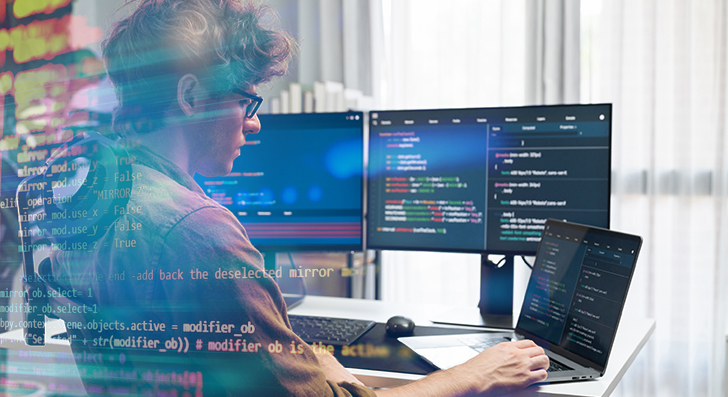
Scalability implies your application can manage expansion—a lot more customers, extra facts, plus more website traffic—with no breaking. Like a developer, constructing with scalability in mind will save time and anxiety afterwards. Listed here’s a clear and realistic manual to help you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it should be part of your respective strategy from the start. A lot of purposes fall short every time they expand speedy due to the fact the first design and style can’t tackle the additional load. As being a developer, you might want to Consider early regarding how your program will behave stressed.
Begin by coming up with your architecture to become flexible. Keep away from monolithic codebases exactly where anything is tightly connected. As an alternative, use modular structure or microservices. These patterns crack your app into smaller sized, impartial pieces. Each and every module or company can scale on its own without having impacting The complete system.
Also, take into consideration your databases from working day 1. Will it need to have to take care of a million customers or maybe 100? Pick the proper sort—relational or NoSQL—dependant on how your information will expand. Prepare for sharding, indexing, and backups early, Even when you don’t have to have them however.
An additional critical place is to prevent hardcoding assumptions. Don’t compose code that only performs underneath latest disorders. Consider what would materialize Should your person base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use structure styles that aid scaling, like information queues or party-driven techniques. These help your app handle much more requests without having receiving overloaded.
If you Create with scalability in your mind, you're not just getting ready for achievement—you might be lessening upcoming complications. A properly-planned method is easier to take care of, adapt, and improve. It’s greater to arrange early than to rebuild afterwards.
Use the best Database
Choosing the ideal databases is actually a important Element of making scalable purposes. Not all databases are designed precisely the same, and using the wrong you can slow you down or even bring about failures as your app grows.
Start by knowledge your details. Could it be highly structured, like rows in a very table? If Certainly, a relational databases like PostgreSQL or MySQL is an efficient fit. These are definitely solid with associations, transactions, and consistency. In addition they help scaling techniques like examine replicas, indexing, and partitioning to handle additional visitors and details.
Should your data is much more flexible—like person activity logs, merchandise catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at handling massive volumes of unstructured or semi-structured details and may scale horizontally more simply.
Also, consider your go through and produce patterns. Will you be doing a great deal of reads with much less writes? Use caching and skim replicas. Have you been handling a large generate load? Consider databases that could handle large generate throughput, or even occasion-dependent details storage techniques like Apache Kafka (for temporary knowledge streams).
It’s also smart to Feel in advance. You may not require State-of-the-art scaling features now, but selecting a databases that supports them means you gained’t have to have to switch later.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your facts based upon your obtain styles. And usually check databases general performance when you mature.
In short, the right databases depends upon your app’s structure, speed needs, And exactly how you count on it to increase. Just take time to choose properly—it’ll save a lot of trouble afterwards.
Improve Code and Queries
Rapid code is essential to scalability. As your app grows, each and every tiny delay adds up. Improperly published code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s vital that you Develop efficient logic from the beginning.
Get started by producing clear, straightforward code. Steer clear of repeating logic and take away nearly anything unwanted. Don’t select the most complicated Alternative if an easy a single functions. Maintain your functions shorter, targeted, and easy to check. Use profiling equipment to locate bottlenecks—sites the place your code will take too extensive to run or employs a lot of memory.
Subsequent, check out your databases queries. These frequently gradual factors down over the code alone. Ensure each question only asks for the information you actually have to have. Stay away from SELECT *, which fetches almost everything, and rather pick out particular fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly throughout huge tables.
For those who recognize the exact same information currently being asked for repeatedly, use caching. Keep the results temporarily working with tools like Redis or Memcached and that means you don’t need to repeat high priced functions.
Also, batch your database operations once you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and can make your application much more productive.
Make sure to exam with huge datasets. Code and queries that get the job done fine with 100 information may possibly crash if they have to handle 1 million.
In brief, scalable apps are quickly apps. Keep the code limited, your queries lean, and use caching when essential. These techniques enable your software continue to be sleek and responsive, at the same time as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to deal with a lot more consumers and a lot more website traffic. If all the things goes by means of one particular server, it is going to promptly turn into a bottleneck. That’s wherever load balancing and caching can be found in. These two equipment aid keep your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout multiple servers. Instead of one server doing many of the do the job, the load balancer routes people to diverse servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can ship traffic to the others. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing data quickly so it could be reused swiftly. When customers ask for precisely the same details once again—like a product site or a profile—you don’t should fetch it through the database anytime. You'll be able to serve it through the cache.
There are two popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) retailers details in memory for rapidly obtain.
two. Consumer-facet caching (like browser caching or CDN caching) merchants static data files near to the person.
Caching lowers databases load, enhances velocity, and helps make your application a lot more efficient.
Use caching for things which don’t modify typically. And usually make certain your cache is current when information does transform.
In a nutshell, load balancing and caching are very simple but effective applications. Together, they help your application deal with additional customers, keep speedy, and recover from challenges. If you propose to develop, you require both.
Use Cloud and Container Equipment
To make scalable applications, you may need applications that let your app increase conveniently. That’s exactly where cloud platforms and containers are available in. They offer you flexibility, minimize set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy hardware or guess long term capacity. When site visitors will increase, you could add more resources with just a few clicks or immediately utilizing auto-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms also provide providers like managed databases, storage, load balancing, and safety resources. You'll be able to give attention to creating your app as an alternative to taking care of infrastructure.
Containers are One more crucial Instrument. A check here container packages your application and anything it should run—code, libraries, settings—into a person device. This causes it to be simple to maneuver your application among environments, out of your notebook to your cloud, without the need of surprises. Docker is the preferred Device for this.
When your application employs numerous containers, tools like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it quickly.
Containers also help it become simple to different areas of your app into services. You may update or scale elements independently, which is great for performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale quick, deploy quickly, and Recuperate promptly when issues transpire. If you'd like your application to develop devoid of boundaries, start employing these applications early. They conserve time, lower danger, and allow you to stay focused on constructing, not correcting.
Keep track of Anything
In the event you don’t keep an eye on your software, you received’t know when issues go Erroneous. Checking helps you see how your application is performing, place difficulties early, and make better decisions as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and expert services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—keep track of your app also. Control just how long it will require for people to load web pages, how frequently glitches transpire, and where by they manifest. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s going on within your code.
Arrange alerts for essential issues. For instance, In case your response time goes above a Restrict or simply a services goes down, you need to get notified immediately. This helps you fix issues speedy, normally in advance of end users even observe.
Monitoring is also practical after you make improvements. In case you deploy a fresh feature and find out a spike in problems or slowdowns, you'll be able to roll it back in advance of it brings about actual damage.
As your application grows, targeted visitors and knowledge improve. Without checking, you’ll skip indications of difficulties until finally it’s too late. But with the appropriate equipment in place, you keep in control.
Briefly, monitoring can help you maintain your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about understanding your process and making sure it really works nicely, even stressed.
Final Thoughts
Scalability isn’t just for significant firms. Even small apps have to have a powerful Basis. By creating thoroughly, optimizing wisely, and using the ideal equipment, you could Construct applications that grow easily without the need of breaking under pressure. Start off compact, Feel major, and build wise.